You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
Add support for shadowColor on Android (API >= 28) (#28650)
Summary:
This PR adds support for the `shadowColor` style on Android.
This is possible as of Android P using the `setOutlineAmbientShadowColor` and `setOutlineSpotShadowColor` View methods. The actual rendered color is a multiplication of the color-alpha, shadow-effect and elevation-value.
## Changelog
`[Android] [Added] - Add support for shadowColor on API level >= 28`
Pull Request resolved: #28650
Test Plan:
- Only execute code on Android P
- Added Android `BoxShadow` tests to RNTester app
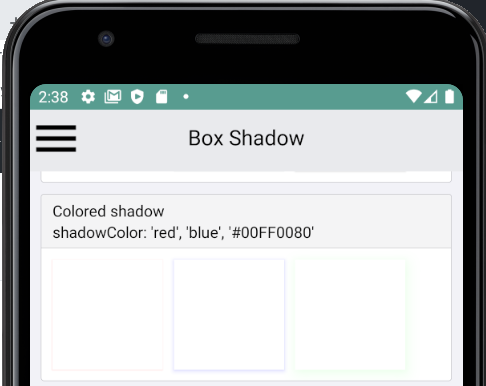
Reviewed By: mdvacca
Differential Revision: D21125479
Pulled By: shergin
fbshipit-source-id: 14dcc023977d7a9d304fabcd3c90bcf34482f137
0 commit comments