You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
Export the DevSettings module, add addMenuItem method (#25848)
Summary:
I wanted to configure the RN dev menu without having to write native code. This is pretty useful in a greenfield app since it avoids having to write a custom native module for both platforms (and might enable the feature for expo too).
This ended up a bit more involved than planned since callbacks can only be called once. I needed to convert the `DevSettings` module to a `NativeEventEmitter` and use events when buttons are clicked. This means creating a JS wrapper for it. Currently it does not export all methods, they can be added in follow ups as needed.
## Changelog
[General] [Added] - Export the DevSettings module, add `addMenuItem` method
Pull Request resolved: #25848
Test Plan:
Tested in an app using the following code.
```js
if (__DEV__) {
DevSettings.addMenuItem('Show Dev Screen', () => {
dispatchNavigationAction(
NavigationActions.navigate({
routeName: 'dev',
}),
);
});
}
```
Added an example in RN tester
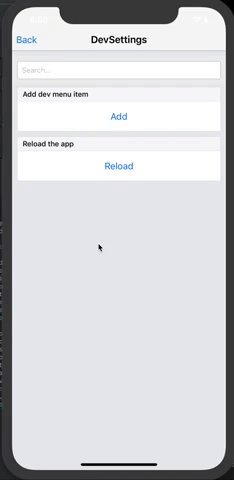
Differential Revision: D17394916
Pulled By: cpojer
fbshipit-source-id: f9d2c548b09821c594189d1436a27b97cf5a5737
0 commit comments