You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
Summary:
Motivation is to support ripple radius just like in TouchableNativeFeedback, plus borderless attribute. See #28009 (comment)
In the current form this means user needs to pass an `android_ripple` prop which is an object of this shape:
```
export type RippleConfig = {|
color?: ?ColorValue,
borderless?: ?boolean,
radius?: ?number,
|};
```
Do we want to add methods that would create such config objects - https://facebook.github.io/react-native/docs/touchablenativefeedback#methods ?
## Changelog
[Android] [Added] - support borderless and custom ripple radius on Pressable
Pull Request resolved: #28156
Test Plan:
Tested locally in RNTester. I noticed that when some content is rendered after the touchables, the ripple effect is "cut off" by the boundaries of the next view. This is not specific to Pressable, it happens to TouchableNativeFeedback too but I just didn't notice it before in #28009. As it is an issue of its own, I didn't investigate that.
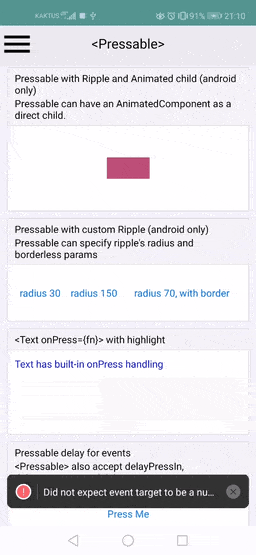
I changed the Touchable example slightly too (I just moved the "custom ripple radius" up to show the "cutting off" issue), so just for completeness:
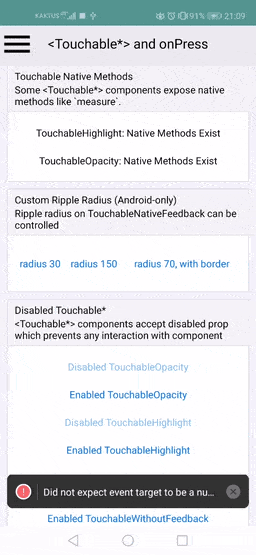
Reviewed By: yungsters
Differential Revision: D20071021
Pulled By: TheSavior
fbshipit-source-id: cb553030934205a52dd50a2a8c8a20da6100e23f
0 commit comments